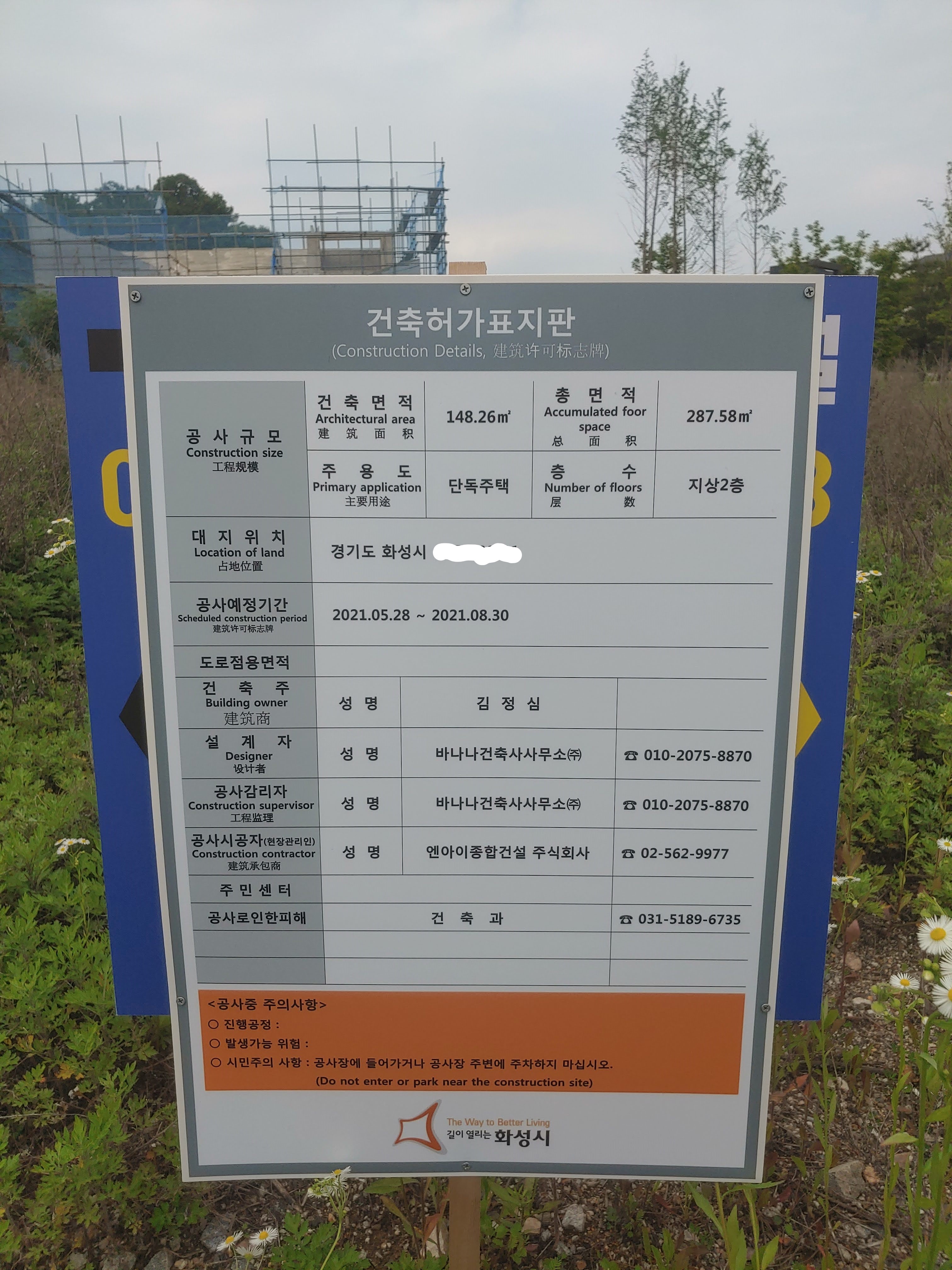
시청에 착공신고를 하기 위해서 건축허가표지판을 세우고, 사진을 찍어 세움터에 제출해야 한다.
시청에 착공신고를 하기 위해서 건축허가표지판을 세우고, 사진을 찍어 세움터에 제출해야 한다.
착공을 위한 첫걸음을 완료했다.
경계복원측량은 인접한 대지와의 경계를 정확하게 측정하기 위해 꼭 필요하며, 한국국토정보공사(LX)에 전화로 신청하거나, 인터넷으로 신청할 수 있다.
1장. 당신은 투자에 있어, 안전 지향형인가? 위험 선호형인가? 왜 차이가 있는가? 이는 성인기 초기의 경험에 의해 크게 좌우된다. 지능도 교육도 아닌, 언제/어디서 태어났는가 하는 우연에 좌우될 뿐이다.
2장. 성공한 사람이 있고, 실패한 사람이 있다. 멋있게 대담했다. 바보같이 무모했다. 어떠한 결과가 100% 노력이나 의사 결정으로 이루어진다고 생각하면 안 된다. 어느 순간 행운이 올지 리스크가 올진 아무도 알 수 없다.
3장. 자신에게 해야 하는 질문 1. 얼마나 더 벌고 싶은가? 2. 누군가와 비교하고 있는가? 3. 충분하다고 느끼는가? 4. 돈보다 중요한 것은 무엇인가?
4장. 시간이 너희를 부유케 하리리, 사람들은 최고의 수익률을 원한다. 최고의 수익률이 아니라 꾸준한 수익률을 오랫동안 유지하는 것이 중요하다. 시간의 힘이 복리의 힘이 중요하다.
5장. 부자가 될 것인가, 부자로 남을 것인가, 누구나 부자가 될 수 있지만, 하지만 모두 부자로 남지는 않는다. 살아남는 것, 생존이 중요하다.
6장. 꼬리가 몸통을 흔든다, 날씨가 항상 좋지는 않다. 비즈니스와 투자도 마찬가지다. 100% 이기는 것이 중요한 것이 아니라, 이길 때 크게 이기고, 질 때 작게 지는 것이 중요하다.
7장. ‘돈이 있다’라는 것의 의미, 내 시간을 내 뜻대로 쓸 수 있다는 게 돈이 주는 가장 큰 배당금이다. 내가 저축하여 한 푼 한 푼 모은 돈은 나의 미래 한 조각을 소유하는 것과 같다.
8장. 페라리가 주는 역설, 사람들은 당신의 차에만 감탄할 뿐이고 당신의 물건을 보고 당신을 존경하지는 않는다.
9장. 부의 정의, 겉으로 드러나는 것으로 그 사람의 성공을 가늠한다. 부란 눈으로 보이는 것으로 바꾸지 않은 것이다. 부자가 되는 길은 가지고 있는 돈을 쓰지 않는 것이다. 소비 부자 대 자산 부자
10장. 소비가 효율적이면 투자 수익률 혹은 소득이 적어도 된다. 저축률을 높일 필요가 있는데(소비를 줄여야 한다) 저축을 늘리는 방법은 겸손을 늘리는 것이다. 사람들에게 보여주려는 행위를 하지 말아라. 저축은 위험에 대한 대비책이고 내 시간을 내 마음대로 할 수 있는 방법이 될 수 있다. 내 뜻대로 쓸 수 있는 시간, 내 마음대로 할 수 있는 선택권을 더 많이 갖는 것은 세상에서 가장 가치 있는 화폐 중 하나이다.
11장. 철저히 이성적이기보다는 적당히 합리적인 수준의 목표로 삼는 것이 좋다. 사람들은 수학적으로 최적의 전략을 원하지 않는다. 밤잠을 잘 잘 수 있는 전략을 원한다. 숫자에 기반한 이성적인 전략으로만 시장을 상대하기 어렵다.
12장. 한 번도 일어난 적이 없는 일들은 언제나 일어난다. 역사가의 오류. 과거로 미래를 예단할 수 없다. 과거에 의존하면 미래를 바꿔놓을 이례적인 일들을 무시할 가능성이 크다. 이론과 공식을 여러 차례 개선했다. 예측이 가능한 건 예측 불가능한 일들이 생긴다는 것뿐이다.
13장. 안전마진은 확실성이 아니라 “행복해지는 최선의 길은 목표를 낮추는 것이다” – 찰리 멍거 95%의 유리함이 있다고 하더라도 5%의 파신 가능성이 위험이 있다면 그 위험을 감수할 가치는 없다.
14장. ‘사람은 변한다.’라는 명제를 투자계획에도 적용하라. 장기 투자 계획을 짜는 것은 어려우니 계속 변화시켜라.
15장. 뭐든 밖에서 보면 쉬워 보인다. 성공적인 투자 역시 밖에서 보면 쉬워 보인다. 하지만 시장에 참여자에게는 어렵다.(투자의 대가는 변동성, 공포 등으로 지불해야 한다). 1. 변동성과 격변을 받아들이며 대가를 지불한다.(장기 투자) 2. 변동성이 적은 중고차 같은 것을 찾는다.(채권) 3. 대가를 치르지 않고 수익을 얻는 전략을 취한다.(단타 같은 투자) 즉 변동성과 공포를 견디지 않고서 수익을 내는 것은 어렵다. 성공 투자의 대가는 가격표처럼 보이지 않기 때문에 수수료처럼 느껴지지 않고 벌금으로 생각한다. 시장 변동성을 벌금이 아니라 수수료처럼 생각하자. 이러면 살아남은 투자자가 될 수 있다. 성공적인 투자에는 대가가 따라붙는다. 변동성 공포 불확실성 후회의 등의 형태로 지불해야한다.
16장. 탐욕스러운 의사 결정을 어떻게 합리화하는가? 나와 다른 게임을 하는 투자자로부터 신호를 읽는다. 단일한 적정 가격은 없다. 투자자들은 서로 다른 시간과 투자 시각을 가지고 있다. 모멘텀이 단기 투자자를 끌어들이고.. 장기 투자자였던 투자자들이 단기 투자자로 돌아서고. 점점 더 많은 단기 투자자가 진입하면서 문제가 발생한다. 나와 다른 게임을 하는 사람들에게 휩쓸리면 안 된다.(투자 및 소비에 대해서) 돈을 많이 쓰고 과시하는 사람은 그것이 필요하기 때문에 그런 게임을 할 수도 있다. 무작정 따라 하면 안 된다. 스스로 물어보라. 30년을 내다보고 있는가? 10년 이내에 현금화할 것인가? 단기 투자자인가?
17장. 비관주의는 낙관주의보다 똑똑하게 들리는 경향이 있다. 기회보다는 위협을 중요시하는 것은 진화적인 요인도 있다. 투자할 때는 성공의 대가가 무엇인지를 생각하고 그 대가를 지불해야 한다. 비관주의의 늪을 명심하라.
18장. 스토리가 세상을 좌우한다. 무엇인가가 간절히 사실이기를 바랄 때, 사실이라고 믿어버린다.(매력적인 허구) 매력적인 허구는 강력해서 아무거나 믿게 만든다. 날씨관을 믿지 않으면서 돌팔이 금융관을 믿는 건 이상하다. 하락을 기대하고 주식을 팔았다면 조그만 나쁜 소식들을 확대하여 해석하게 된다. 내 머릿속의 한정된 모형으로 세상을 이해하고.. 결과적으로 많이 틀린다. 우리는 예측 가능하고 통제 가능한 세상에 살고 있다는 믿음이 필요해서 예측하는 권위자들에게 의지한다.
19장. 겸손을 찾고 연민을 찾아라. 자존심은 줄이고 부를 늘려라. 밤잠을 설치지 않을 방법을 찾아라. 시간을 보는 눈을 넓혀라. 시간은 기다리는 사람에게 결과를 밀어줄 수 있다. 포트폴리오에 일부가 아닌 전체를 보라. 내 시간을 내 뜻대로 하는 데 돈을 써라. 당신이 원할 때 원하는 것을 원하는 사람과 원하는 만큼 할 수 있는 것은 돈이 주는 힘이다. 저축하라. 그냥 저축하라. 성공을 위한 비용은 기꺼이 지출하라. 실수의 여지에 항상 대비하라. 파산하지 않고 게임을 계속 이어갈 수 있다. 리스크를 좋아하라. 그러나 파산할만한 리스크는 회피하라.
20장. 당신은 무엇을 소유하고 있는가? 왜 그것을 소유하고 있는가? 보편적 진리는 없다. 기본적인 원칙은 있다. 저축 전략. 부자가 되는 것이 아니라 독립성을 원한다. 준비되었을 때 내가 하고 싶은 일을 할 수 있는 것이다. 중요한 것은 저축률과 검소한 생활양식이다. 저비용 인덱스 펀드에 모두 투자하고 있다. 높은 저축률과 인내심, 세계 경제가 성과를 창출하리라는 낙관주의의 3가지가 나의 투자 전략이다.
저자는 현대의 전문화 시스템을 기업, 정부, 교육 기관이 편의를 위해서 만든 제도라고 이야기하며, 전문화 시스템은 우리의 생존에도 위협이 될 뿐 아니라 우리가 지적으로나 영적으로 성장하는 데에도 걸림돌이 된다고 이야기하며, 폴리매스를 찾는 지도의 구성 요소는 여섯 가지를 이야기한다. 개성, 호기심, 지능, 다재다능함, 창의성, 통합
개성 : 진짜 자기를 찾는 여정을 마쳐야만 바깥 세계의 삶을 바꾸는 여정을 떠날 수 있다. 자신의 참모습과 잠재성을 발견하는 일은 시행착오가 필요한 과정이므로 가장 좋은 방법은 다양한 일에 도전하며 각 경험에 대한 자신의 반응을 평가하는 것이다. 괴짜로 불리는 이들은 생각하고 행동하는 방식이 그저 대다수의 사람과 다를 뿐 지극히 합리적이고 행복한 사람들이다.
호기심 : 호기심은 폴리매스를 움직이는 원동력으로 우리의 의식과 몸속에 깊이 뿌리내리고 있다. 지식의 원천은 다양하고 목적에 따라 저마다 가치가 있다. 폴리매스라면 다양한 원천에서 지식을 얻어야 하고 그 과정에서 자연스럽게 여러 분야의 지식을 습득하게 된다.
지능 : 머리가 더 좋은 사람은 맡은 역할에 구애받지 않고 더 전략적으로 사고하는 경향이 있다. 폴리매스는 자신의 경험과 지식을 토대로 일반 지능과 비판적 사고 능력을 최대한 활용해 해당 분야의 원리를 이해할 수 있다.
다재다능함 : 다재다능함은 무관해 보이는 여러 영역을 매끄럽게 넘나드는 능력을 뜻한다. 꼭 필요해서 하는 일과 열정으로 하는 일을 번갈아 하면 정신 건강에 좋다. 다재다능성과 적응력, 개발성과 회복탄력성을 배양하는 사고방식, 다시 말해 ‘유연한 사고’를 기를 때 우리는 거의 모든 삶의 영역에서 변화가 증폭되는 21세기에 적응하고 번성할 수 있다.
창의성 : 혁신적인 사람이 보통 사람보다 훨씬 폭넓게 활동하고 보다 다양한 기술을 배양하는 경향이 있다. 폭넓은 기술과 지식, 경험을 갖추고 있으면 더 큰 그림을 보는 능력이 생기고 창의적 혁신을 가능하게 한다. 뛰어난 과학자들은 대체로 다수의 취미를 즐겼다고 밝혔고, 예술은 과학에 영감을 줄 수 있다. 과학과 예술이 둘 사이의 간극을 채우고, 다른 세계로 도약하는 발판 역할을 수행한다.
통합성 : 인간의 타고난 의식은 전일적 관점으로 세계를 볼 때 자연스럽다. 지식의 통합은 “인간 본성의 고귀한 충동을 만족시키고” 또 “지성이 추구할 마땅한 목표를 제공한다.” 통합성은 맥락과 밀접한 관련이 있다. 맥락을 파악하려면 문제와 직간접적으로 관련된 다수의 현상을 고려해야 다차원적이고 전방위적인 분석이 가능하다. 좌반구가 주도하는 사고에서 벗어나 ‘완전한’ 정신을 지향해야 하고, 두 반구를 상호보완적으로 이용하는 방향으로 사고를 전환할 필요가 있다. 이를 위해서는 좌우 반구의 균형 잡힌 사고를 효과적으로 촉진하는 교육 제도와 업무 환경 그리고 이를 뒷받침하는 문화를 조성해야 한다. 인터넷 시대에 정보를 간단하게 접근할 수 있는 세상이다. 비판적 사고를 통해서 지식을 제대로 구성하고 이해하고 이용해야 한다.
저자는 인류 진화에 따르면 사피엔스는 나머지 종과 다르게 인지 혁명을 거쳐 살아남았다고 이야기하며 폴리매스형 인간들이 새로운 인지 혁명을 만들어야 인류의 현재 멸종 위기를 극복할 수 있다고 이야기한다.
사회의 발전은 천재들이 이끈다고 생각하는데, 현재의 교육 시스템은 천재들을 폴리매스가 아닌, 특정 분야의 전문가로 한계를 만드는 것이 아닌가?라는 생각도 들었고, 빌 게이츠와 같은 천재가 있기 때문에 소프트웨어 분야가 이렇게 발전할 수 있었기 때문에, 전문화 시스템이 필요한 것이 아닌가?라는 생각도 하게 되었다. 정답은 알 수 없지만, 개인적으로는 자녀 교육에 있어 저자가 이야기한 여섯 가지에 관심을 가진다면, 천재는 아니더라도 어떤 일을 하더라도 재미있게 할 수 있는 인재가 될 수 있을 것 같다고 생각하고 적용을 해보려고 한다.
레이 커즈와일은 어떤 프로젝트든 그 리더는 폴리매스여야 한다고 주장한다. “한가지 분야에 고도로 전문화된 전문가는 팀원으로 일하면 되고, 팀 리더는 여러 분야 사이에 교량 역할을 할 줄 알아야 한다.”